Project setup and creating a module
Before we write any code, you’ll need to create a snippetbox
directory on your computer to act as the top-level ‘home’ for this project. All the Go code we write throughout the book will live in here, along with other project-specific assets like HTML templates and CSS files.
So, if you’re following along, open your terminal and create a new project directory called snippetbox
anywhere on your computer. I’m going to locate my project directory under $HOME/code
, but you can choose a different location if you wish.
$ mkdir -p $HOME/code/snippetbox
Creating a module
The next thing you need to do is decide on a module path for your project.
If you’re not already familiar with Go modules, you can think of a module path as basically being a canonical name or identifier for your project.
You can pick almost any string as your module path, but the important thing to focus on is uniqueness. To avoid potential import conflicts with other people’s projects or the standard library in the future, you want to pick a module path that is globally unique and unlikely to be used by anything else. In the Go community, a common convention is to base your module paths on a URL that you own.
In my case, a clear, succinct and unlikely-to-be-used-by-anything-else module path for this project would be snippetbox.alexedwards.net
, and I’ll use this throughout the rest of the book. If possible, you should swap this for something that’s unique to you instead.
Once you’ve decided on a unique module path, the next thing you need to do is turn your project directory into a module.
Make sure that you’re in the root of your project directory and then run the go mod init
command — passing in your chosen module path as a parameter like so:
$ cd $HOME/code/snippetbox $ go mod init snippetbox.alexedwards.net go: creating new go.mod: module snippetbox.alexedwards.net
At this point your project directory should look a bit like the screenshot below. Notice the go.mod
file that has been created?
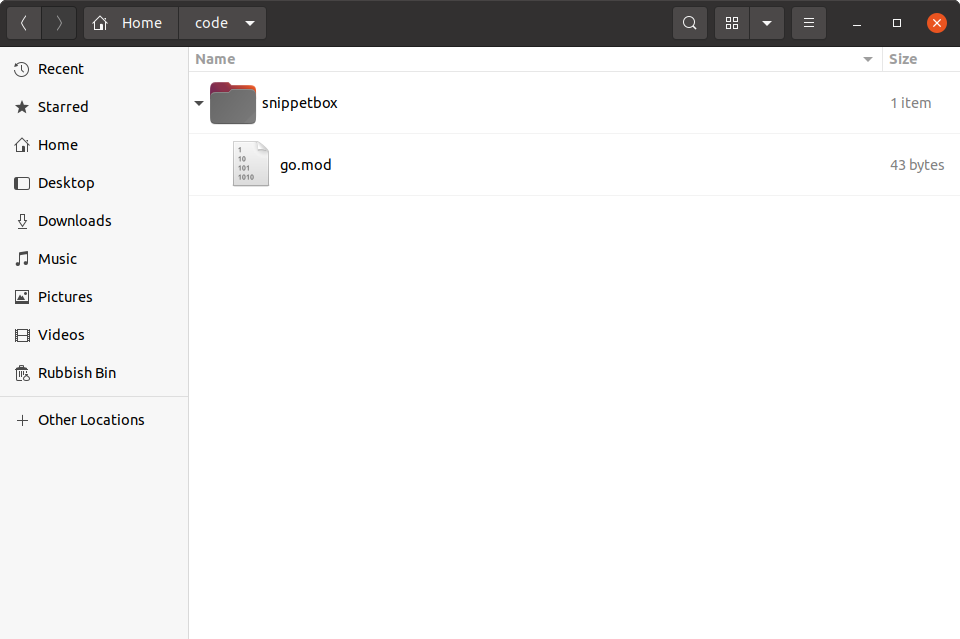
At the moment there’s not much going on in this file, and if you open it up in your text editor it should look like this (but preferably with your own unique module path instead):
module snippetbox.alexedwards.net go 1.24.0
We’ll talk about modules in more detail later in the book, but for now it’s enough to know that when there is a valid go.mod
file in the root of your project directory, your project is a module. Setting up your project as a module has a number of advantages — including making it much easier to manage third-party dependencies, avoid supply-chain attacks, and ensure reproducible builds of your application in the future.
Hello world!
Before we continue, let’s quickly check that everything is set up correctly. Go ahead and create a new main.go
file in your project directory containing the following code:
$ touch main.go
package main import "fmt" func main() { fmt.Println("Hello world!") }
Save this file, then use the go run .
command in your terminal to compile and execute the code in the current directory. All being well, you will see the following output:
$ go run . Hello world!
Additional information
Module paths for downloadable packages
If you’re creating a project which can be downloaded and used by other people and programs, then it’s good practice for your module path to equal the location that the code can be downloaded from.
For instance, if your package is hosted at https://github.com/foo/bar
then the module path for the project should be github.com/foo/bar
.